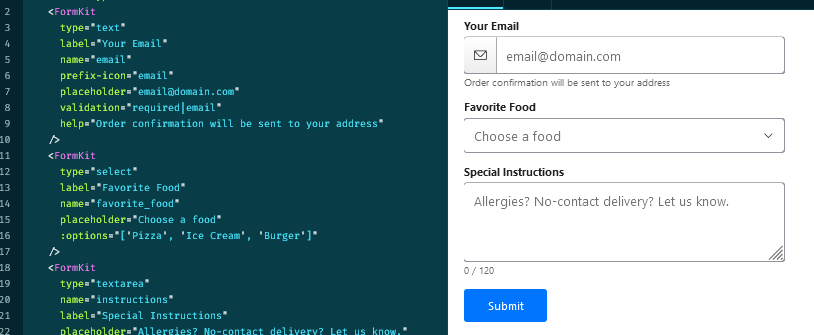
While using the autopopulate plug-in is quick and easy, what are your options if you have a field that doesn't work well as a textbox? For example, if you just wanted to display a label.
Out of the box, Formkit doesn't have just a label control. With just a few extra lines of code, you can create one, though. And doing so will allow the label to be autopopulated with the same plug-in that populates the rest of the Formkit nodes.
Here is one way of creating the Formkit equivalent of a html label element:
<form-kit
id="remarks"
name="remarks"
type="text"
label=" "
value=""
readonly
:outer-class="{
'formkit-outer': false
}"
:inner-class="{
'formkit-inner': false
}"
:label-class="{
'formkit-label': false
}"
:wrapper-class="{
'formkit-wrapper': false
}"
:sections-schema="{
input: { $el: '' },
label: {
children: [
'$label',
{
if: '$value != undefined',
then: {
$el: 'span',
children: '$value',
},
},
],
},
}"
:classes="{
label: 'relative-ns top-1',
}"
>
This is just a normal Formkit input of the input type "text," but any default css is removed and the schema has been changed so that the textbox is removed. This will cause only the Formkit label to be seen. The altered schema is simply mirroring the node value to the Formkit label. One advantage to this setup over a plain html label element is that the Formkit node still has a valid value, even though only the label is being displayed.
If you happen to just need a label, but think the above is too verbose, it is quite easy to populate plain html elements using the same json object being used to autopopulate a Formkit form.
nonFormKitControls(data) {
const inputs = Array.prototype.slice.call(document.querySelectorAll('label'));
Object.keys(data).map(function (dataItem) {
inputs.map(function (inputItem) {
return (inputItem.id === dataItem) ? (inputItem.innerText = data[dataItem]) : false;
});
});
}
},
})
This is simply a method that gets an array of the desired elements, loops through it, and if any of the element ids equal the key of the json object, will assign it the key's value (with labels, we are assigning the value using .innerText. This will change with different inputs.).
The trick here is the same one used with autopopulating Formkit nodes. Your label must have an id that is the same as a key in the json data object.
For display only, this is as easy as modifying a Formkit node to act as a display. The disadvantage here is that if you did need the text of that label to be submitted with a Formkit form, you will have to get it using vanilla javascript and append it to the Formkit data before sending it to the server.
As you can see, either way produces similar results. There isn't a right or wrong way here. Use the one that fits the situation the best.